Cookbook: Turn sentry alerts into notifcations
In this section, we will demonstrate how you can use notifyz to get realtime notification on your phone or desktop of new Sentry alerts !
Prerequisites
- A sentry account
- A notifyz account
1. Setup your notifyz topic
Once you have register to notifyz and choose the plan that fits your needs, go to the topic section and create a new topic.
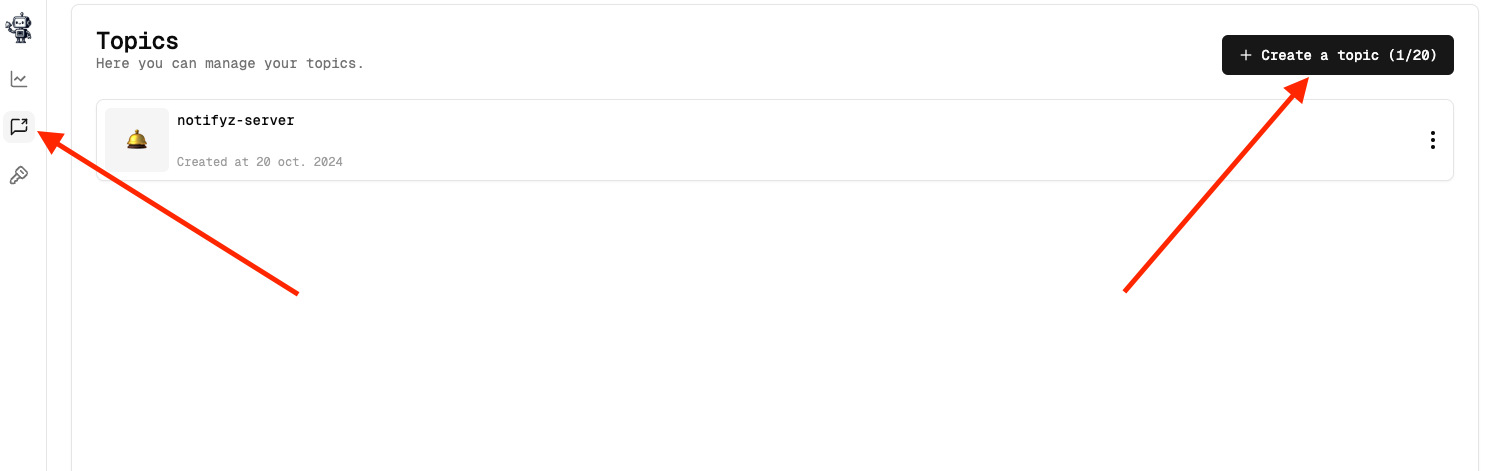
Once you topic is ready, create a "Subscription link" and share it with the devices you want to receive notifications.
2. Setup your sentry integration
-
In sentry go to Setting > Developer Setting > Custom integration
-
Then click "Create New Integration"
-
Choose "Internal Integration"
-
Fill your application information (Name, Webhook URL), enable "Alert Rule Action" (you can edit those information later)
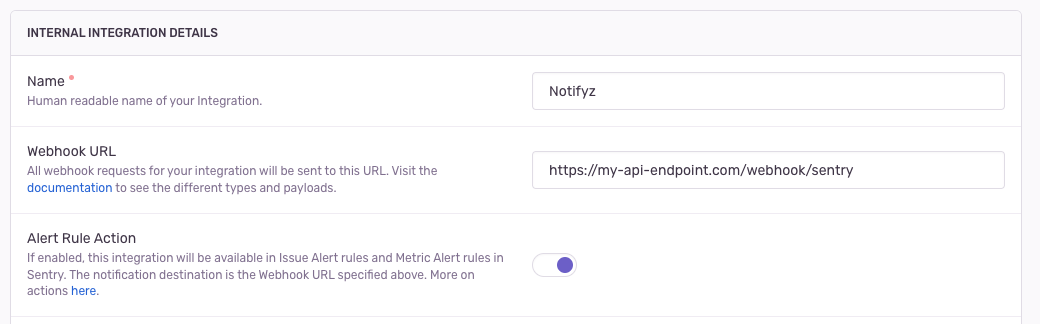
- Give read access to the resource you want to monitor, and select webhook events you want to receive
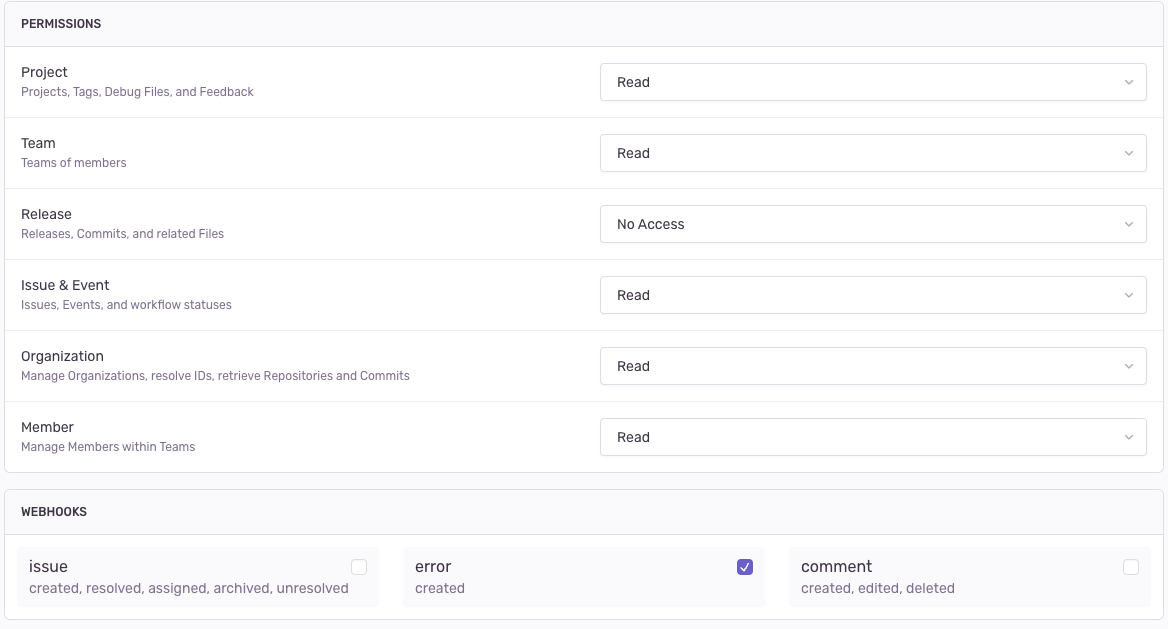
3. Setup webhooks endpoint
Once your notifyz topic and your sentry integration are ready, you can setup your webhooks endpoint.
I personnaly use Cloudflare Workers, but the choice is yours.
-
Go to your notifyz topic, click on the 3 dots icon then "Integrate"
-
Follow the intstuction, then grab the code example including your topic endpoint and your api key
-
Go to you sentry integration, and copy your "Client Secret"
PS: Has a security advice, all thoses information should be store in a secure context and never be commited to your codebase.
1import { createHmac } from "node:crypto";
2
3
4const SENTRY_CLIENT_SECRET = "YOUR_SENTRY_CLIENT_SECRET";
5const NOTIFYZ_API_KEY = "YOUR_NOTIFYZ_API_KEY";
6const NOTIFYZ_TOPIC_ENDPOINT = "https://api.notifyz.dev/topics/YOUR_TOPIC_ID/notify";
7
8const hmac = createHmac("sha256", SENTRY_CLIENT_SECRET);
9hmac.update(await request.clone().text(), "utf8");
10
11const digest = hmac.digest("hex");
12
13if (digest !== request.headers.get("sentry-hook-signature")) {
14 throw new Error("Invalid signature");
15}
16
17const body = await request.json();
18
19const response = await fetch(NOTIFYZ_TOPIC_ENDPOINT, {
20 headers: {
21 "Content-Type": "application/json",
22 "X-Api-Key": env.NOTIFYZ_API_KEY
23 },
24 method: "POST",
25 body: JSON.stringify({
26 title: body.data.error.title.slice(0, 100),
27 body: body.data.error.web_url,
28 imageURL: "https://x5h8w2v3.rocketcdn.me/wp-content/uploads/2023/06/Logo-Sentry.png",
29 redirectURL: body.data.error.web_url,
30 })
31});
32
33if (!response.ok) {
34 throw new Error(await response.text());
35}
36
37
38return new Response('Ok');
The above code example will show you a basic process of:
- Verifying the sentry webhook signature
- Exracting information from the sentry webhook payload
- Sending a notification to your notifyz topic
Some part of this example will probably need to be changed to fit your needs, but it should give you a good starting point.